Javascript Subtleties: ?? vs ||
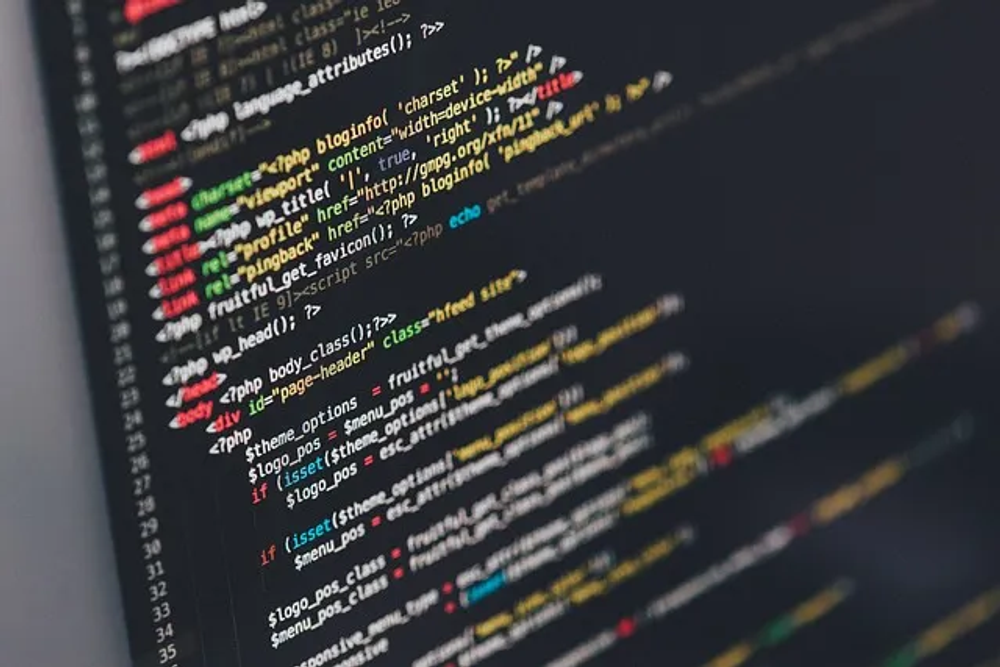
In JavaScript, ??
and ||
are two operators that serve distinct purposes, but are often confused with each other. In this post, we’ll dive into the differences between these two operators and explore when to use each one.
The ||
Operator (Logical OR)
The ||
operator is a logical OR operator. It returns the value of the first operand if it’s truthy, and the value of the second operand if the first one is falsy.
Here’s how it works:
- If the first operand is truthy (i.e.,
true
, a non-empty string, a non-zero number, an object, etc.), the expression returns the value of the first operand. - If the first operand is falsy (i.e.,
false
,0
,""
,null
,undefined
, etc.), the expression returns the value of the second operand.
Example
Copyconst foo = null; const bar = 'default'; console.log(foo || bar); // Output: "default"
In this example, foo
is null
, which is falsy, so the expression returns the value of bar
, which is "default"
.
The ??
Operator (Nullish Coalescing)
The ??
operator is a nullish coalescing operator, introduced in JavaScript in 2020 (ECMAScript 2020). It returns the first operand if it’s not null or undefined, and the second operand if the first one is null or undefined.
Here’s how it works:
- If the first operand is not null or undefined, the expression returns the value of the first operand.
- If the first operand is null or undefined, the expression returns the value of the second operand.
Example
Copyconst foo = null; const bar = 'default'; console.log(foo ?? bar); // Output: "default"
In this example, foo
is null
, which is nullish, so the expression returns the value of bar
, which is "default"
.
Key Differences
Here are the main differences between ||
and ??
:
||
checks for falsiness (i.e.,false
,0
,""
,null
,undefined
, etc.), while??
checks specifically for nullish values (i.e.,null
orundefined
).||
returns the first truthy value, while??
returns the first non-nullish value.
When to Use Each Operator
When to Use ||
- When you want to provide a default value if the first operand is falsy.
- When you want to chain multiple conditions together (e.g.,
a || b || c
).
When to Use ??
- When you want to provide a default value if the first operand is null or undefined.
- When you want to simplify code that checks for null or undefined values.
In summary, ||
is a more general-purpose operator for handling falsy values, while ??
is a more specific operator for handling nullish values. By understanding the differences between these two operators, you can write more concise and efficient code.