Docker and GitHub Container Registry (GHCR) Quickstart
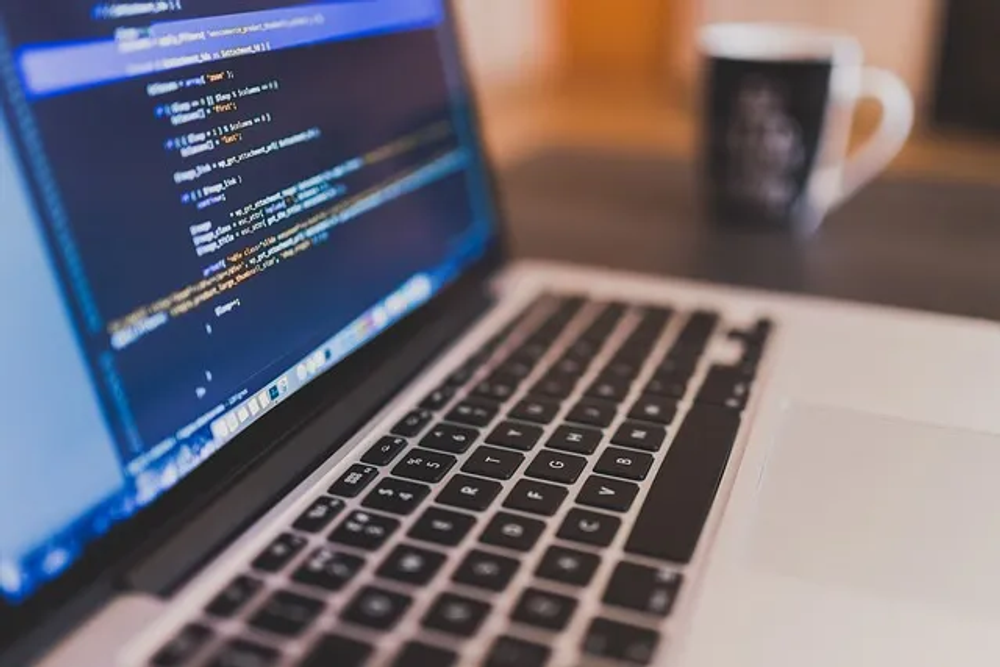
Introduction
GitHub Container Registry (GHCR) is a container image registry that allows you to store and manage Docker images directly in your GitHub repositories. This makes it easier to integrate your container images with your codebase and CI/CD pipelines. In this guide, you’ll learn how to authenticate to GHCR, push your Docker image, and use it in your projects with Docker Compose.
Step 0: Prerequisites
Before you begin, make sure you have the following prerequisites:
- A GitHub account
- Docker Engine installed on your local machine
- Basic knowledge of Docker and containerization concepts
You also need to have a Docker image to push to GHCR. If you don’t have an image yet, you can build one using a Dockerfile
in your project directory.
Here’s a simple example for a Flask app:
Copy# app.py from flask import Flask app = Flask(__name__) @app.route('/') def hello_world(): return 'Hello, World!' if __name__ == '__main__': app.run(host='0.0.0.0', port=8000)
Copy# Dockerfile FROM python:3.12-slim WORKDIR /usr/src/app COPY ./ /usr/src/app RUN pip install Flask==3.0.3 EXPOSE 8000 CMD ["python", "app.py"]
Step 1: Create a GitHub Personal Access Token
-
Login to your GitHub account.
-
Go to Settings → Developer Settings → Personal access tokens. → Tokens (classic)
- Click Generate new token.
-
Give your token a name, select an expiration, and check
write:packages
,read:packages
, anddelete:packages
to push, pull, and delete images. -
Generate the token and make sure to copy it — you won’t be able to see it again!
Step 2: Authenticate to GitHub Package Registry
Login to Docker using your GitHub username and the token you just created as the password:
Copyexport GITHUB_TOKEN=your-personal-access-token echo $GITHUB_TOKEN | docker login ghcr.io -u USERNAME --password-stdin
Replace USERNAME
with your GitHub username.
Step 3: Tag Your Docker Image
- Build your Docker image with:
Copydocker build -t your-image-name .
- Tag the built image to match the GitHub Container Registry format:
Copydocker tag your-image-name ghcr.io/USERNAME/REPOSITORY/YOUR-IMAGE-NAME:TAG
Replace USERNAME
, REPOSITORY
, YOUR-IMAGE-NAME
, and TAG
accordingly. For example, if your username is john
, repository is myapp
, the image name you want is app
, and the tag is latest
, the command would be:
Copydocker tag your-image-name ghcr.io/john/myapp/app:latest
Step 4: Push the Image to GitHub Registry
Now you are ready to push your container to GHCR! Push your Docker image using:
Copydocker push ghcr.io/USERNAME/REPOSITORY/YOUR-IMAGE-NAME:TAG
Step 5: Use the Image
Pull the Image
Your image is now stored in GitHub Container Registry and can be pulled to any machine.
Once you have authenticated to Docker, you can pull the image using:
Copydocker pull ghcr.io/USERNAME/REPOSITORY/YOUR-IMAGE-NAME:TAG
Use the Image in Docker-Compose
If you are using docker-compose
to orchestrate containers, you can reference the image in your docker-compose.yml
file like this:
Copy# docker-compose.yml services: your-service: image: ghcr.io/USERNAME/REPOSITORY/YOUR-IMAGE-NAME:TAG ports: - "8000:8000"
Conclusion
Congratulations on successfully navigating the steps to push and pull Docker containers using the GitHub Container Registry (GHCR)! This guide has provided you with a fundamental understanding of how to integrate Docker with GHCR to streamline the management and deployment of your container images. By using GHCR, you benefit from enhanced collaboration, version control, and security features directly integrated with your GitHub workflows.
Remember, the ability to store and manage Docker images alongside your codebase simplifies development processes and improves the consistency across different environments. Whether you are developing locally, deploying to testing environments, or releasing to production, a container registry like GHCR can serve as a reliable and efficient hub for your containerized applications.
Keep exploring and refining your container management strategies to fully leverage the power of Docker and GitHub in your CI/CD pipelines. By continuously improving your DevOps practices, you can achieve more robust, scalable, and maintainable software systems.